23. June 2021
Lucid Slugify updated to work with V5
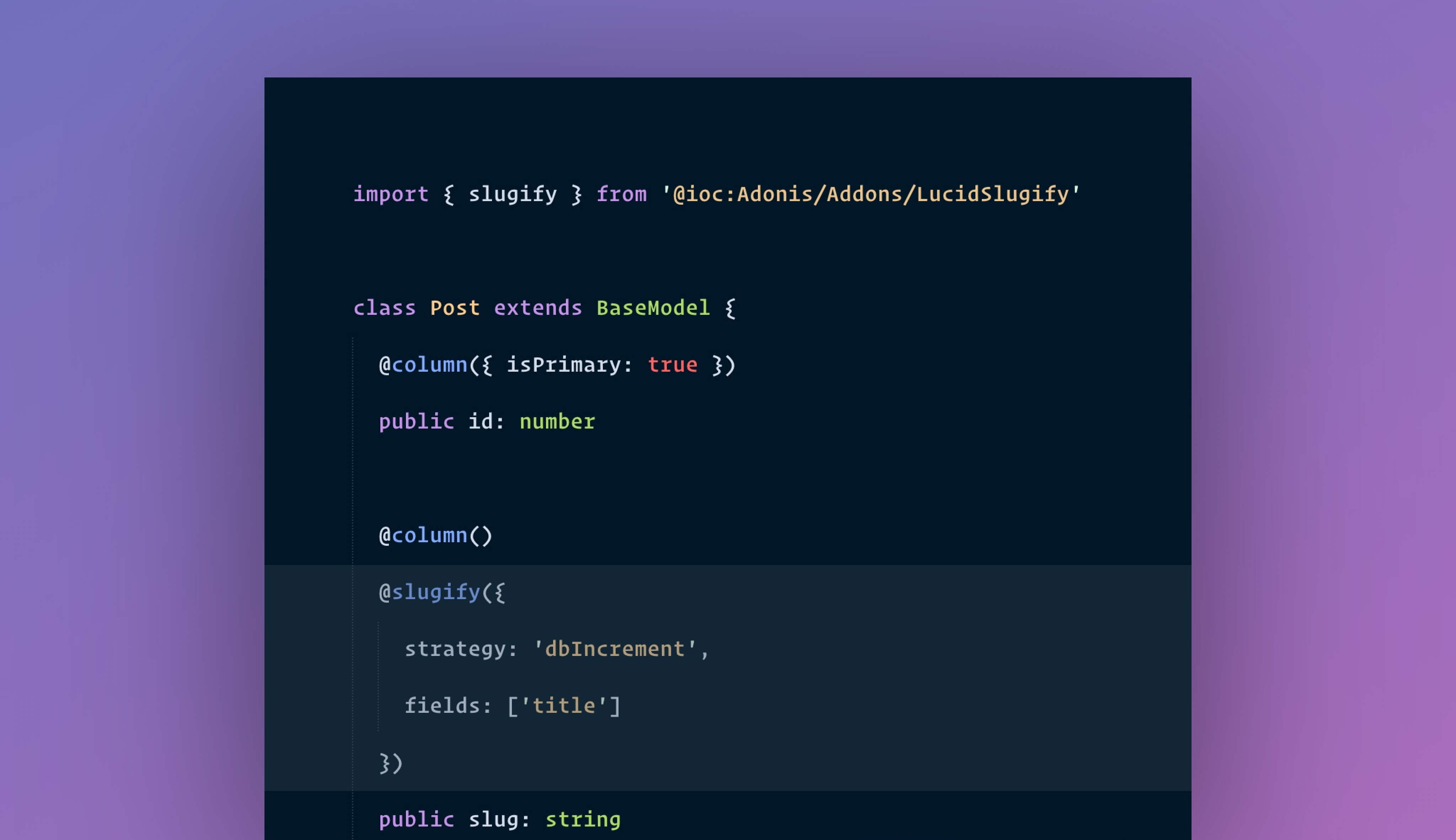
Lucid Slugify for AdonisJS v5
@adonisjs/[email protected]
is ported over to work with AdonisJS v5
Lucid Slugify allows to generate slugs for all kinds of database columns in Lucid models.
It comes with powerful slugification strategies and really flexible configuration. In general creating slugs manually is quite easy, things get more complex when need to generate slugs while avoiding duplicates. For example when working with e-commerce product or blog post URLs
Using Slugify
Since auto generating slugs are not required for all projects it’s standalone AdonisJS module and not included into Lucid core.
To use Slugify it has to be first installed:
npm i @adonisjs/lucid-slugify
And then provider registered, which can be done with:
node ace configure @adonisjs/lucid-slugify
Slugify comes with TypeScript decorator that can be imported and added to Lucid model columns
import { BaseModel, column } from '@ioc:Adonis/Lucid/Orm'
import { slugify } from '@ioc:Adonis/Addons/LucidSlugify'
class Product extends BaseModel {
@column({ isPrimary: true })
public id: number
// Generate `url` automatically based on `company` and `name` fields
// and use database incrementing strategy
@column()
@slugify({
strategy: 'dbIncrement',
fields: ['company', 'name']
})
public url: string
@column()
public name: string
@column()
public company: string
}
Now we can use that model to generate multiple products and don’t have to worry about generating URL slug manually
const tool1 = await Product.create({
name: 'hammer',
company: 'DeWALT'
})
const tool2 = await Product.create({
name: 'hammer',
company: 'DeWALT'
})
console.log(tool1);
/*
{
name: 'hammer',
company: 'DeWALT',
url: 'dewalt-hammer'
}
*/
console.log(tool2);
/*
{
name: 'hammer',
company: 'DeWALT',
url: 'dewalt-hammer-1'
}
*/
Notice how tool2
got -1
added to the end. That’s because dbIncrement
strategy checks for duplicate slugs from database before generating new one.
In case of slug collisions it increments number in the end
Lucid-Slugify has quite some different configuration options. Read more about them all in documentation